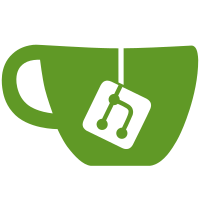
15 changed files with 81 additions and 205 deletions
@ -0,0 +1,15 @@ |
|||
|
|||
|
|||
//TODO: do this with files
|
|||
#define ATTRACTOR_FILE "attractors/testPolynomial.stf" |
|||
|
|||
#ifdef UNI_BUILD |
|||
#warning Building for the RU, are you sure? |
|||
#define WIDTH 8000 |
|||
#define HEIGHT 8000 |
|||
#define ITERATIONS 800000000 |
|||
#else |
|||
#define WIDTH 800 |
|||
#define HEIGHT 800 |
|||
#define ITERATIONS 200000 |
|||
#endif |
Reference in new issue