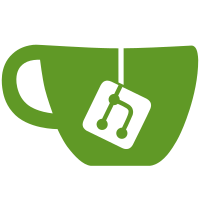
16 changed files with 217 additions and 71 deletions
@ -0,0 +1,43 @@ |
|||
//
|
|||
// Random.hpp
|
|||
// AwesomeAttract0r
|
|||
//
|
|||
// Created by Joshua Moerman on 12/28/11.
|
|||
// Copyright (c) 2011 Vadovas. All rights reserved.
|
|||
//
|
|||
|
|||
#ifndef AwesomeAttract0r_Random_hpp |
|||
#define AwesomeAttract0r_Random_hpp |
|||
|
|||
#include <vector> |
|||
|
|||
// capital because random() was already defined :(
|
|||
namespace Random { |
|||
// used as dummy paramter
|
|||
struct parameters {}; |
|||
|
|||
/*!
|
|||
All uniform distributions are inclusive (ie [min, max]). |
|||
*/ |
|||
template <typename T> |
|||
typename std::enable_if<std::is_arithmetic<T>::value && !std::is_integral<T>::value, T>::type uniform(T min, T max){ |
|||
return min + (rand() / (T) RAND_MAX) * (max - min); |
|||
} |
|||
|
|||
template <typename T> |
|||
typename std::enable_if<std::is_arithmetic<T>::value && std::is_integral<T>::value, T>::type uniform(T min, T max){ |
|||
return min + (rand() % (max - min + 1)); |
|||
} |
|||
|
|||
template <typename T> |
|||
std::vector<T> make_vector(T min, T max, size_t number_of_elements){ |
|||
std::vector<T> ret; |
|||
for (size_t i = 0; i < number_of_elements; ++i) { |
|||
ret.push_back(uniform(min, max)); |
|||
} |
|||
return ret; |
|||
} |
|||
|
|||
} // namespace random
|
|||
|
|||
#endif |
@ -0,0 +1,99 @@ |
|||
//
|
|||
// stf_ext.hpp
|
|||
// AwesomeAttract0r
|
|||
//
|
|||
// Created by Joshua Moerman on 12/28/11.
|
|||
// Copyright (c) 2011 Vadovas. All rights reserved.
|
|||
//
|
|||
|
|||
#ifndef AwesomeAttract0r_stf_ext_hpp |
|||
#define AwesomeAttract0r_stf_ext_hpp |
|||
|
|||
#include <sstream> |
|||
#include <list> |
|||
#include <vector> |
|||
#include <array> |
|||
|
|||
#include "stf.hpp" |
|||
|
|||
namespace std { |
|||
template <typename T> |
|||
std::string to_string(T const & x){ |
|||
std::stringstream ss; |
|||
ss << x; |
|||
return ss.str(); |
|||
} |
|||
} |
|||
|
|||
namespace stfu { |
|||
|
|||
// Prototypes
|
|||
#define to_stf_container_proto(x) \ |
|||
template <typename T> \ |
|||
typename std::enable_if<std::is_fundamental<T>::value, node>::type to_stf(x<T> const & array); \ |
|||
template <typename T> \ |
|||
typename std::enable_if<!std::is_fundamental<T>::value, node>::type to_stf(x<T> const & array); |
|||
|
|||
to_stf_container_proto(std::vector) |
|||
to_stf_container_proto(std::list) |
|||
|
|||
#undef to_stf_container_proto |
|||
|
|||
template <typename T, size_t N> |
|||
typename std::enable_if<std::is_fundamental<T>::value, node>::type to_stf(std::array<T, N> const & array); |
|||
template <typename T, size_t N> |
|||
typename std::enable_if<!std::is_fundamental<T>::value, node>::type to_stf(std::array<T, N> const & array); |
|||
template <typename T> |
|||
node to_stf(T const & x); |
|||
|
|||
// implementations
|
|||
#define to_stf_container(x) \ |
|||
template <typename T> \ |
|||
typename std::enable_if<std::is_fundamental<T>::value, node>::type to_stf(x<T> const & array) { \ |
|||
node node; \ |
|||
for (auto it = array.cbegin(); it != array.cend(); ++it){ \ |
|||
node.addValue() = std::to_string(*it); \ |
|||
} \ |
|||
return node; \ |
|||
} \ |
|||
\ |
|||
template <typename T> \ |
|||
typename std::enable_if<!std::is_fundamental<T>::value, node>::type to_stf(x<T> const & array) { \ |
|||
node node; \ |
|||
for (auto it = array.cbegin(); it != array.cend(); ++it){ \ |
|||
node.addChild() = to_stf(*it); \ |
|||
} \ |
|||
return node; \ |
|||
} |
|||
|
|||
to_stf_container(std::vector) |
|||
to_stf_container(std::list) |
|||
|
|||
#undef to_stf_container |
|||
|
|||
template <typename T, size_t N> |
|||
typename std::enable_if<std::is_fundamental<T>::value, node>::type to_stf(std::array<T, N> const & array) { |
|||
node node; |
|||
for (auto it = array.cbegin(); it != array.cend(); ++it){ |
|||
node.addValue() = std::to_string(*it); |
|||
} |
|||
return node; |
|||
} |
|||
|
|||
template <typename T, size_t N> |
|||
typename std::enable_if<!std::is_fundamental<T>::value, node>::type to_stf(std::array<T, N> const & array) { |
|||
node node; |
|||
for (auto it = array.cbegin(); it != array.cend(); ++it){ |
|||
node.addChild() = to_stf(*it); |
|||
} |
|||
return node; |
|||
} |
|||
|
|||
template <typename T> |
|||
node to_stf(T const & x){ |
|||
return x.to_stf(); |
|||
} |
|||
|
|||
} // namespace stfu
|
|||
|
|||
#endif |
Reference in new issue