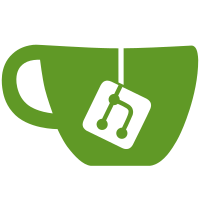
9 changed files with 154 additions and 86 deletions
@ -0,0 +1,48 @@ |
|||
//
|
|||
// output.hpp
|
|||
// AwesomeAttract0r
|
|||
//
|
|||
// Created by Joshua Moerman on 1/8/12.
|
|||
// Copyright (c) 2012 Vadovas. All rights reserved.
|
|||
//
|
|||
|
|||
#ifndef AwesomeAttract0r_output_hpp |
|||
#define AwesomeAttract0r_output_hpp |
|||
|
|||
#include "Logger.hpp" |
|||
#include "stf.hpp" |
|||
#include "Random.hpp" |
|||
#include "Image.hpp" |
|||
#include "Tonemapper.hpp" |
|||
|
|||
namespace details { |
|||
template <typename C, typename TM> |
|||
void output(C const & canvas, TM & tonemapper, std::string const & image_path, stfu::node & stf_output){ |
|||
logger.start("Analysing"); |
|||
tonemapper.analyse(canvas); |
|||
logger.stop(); |
|||
|
|||
ImageFormats::png::png_stream image(canvas.template size<0>(), canvas.template size<1>(), image_path + ".png"); |
|||
logger.start("Exporting"); |
|||
tonemapper.process(canvas, image); |
|||
logger.stop(); |
|||
|
|||
stf_output.addChild("tonemapper") = stfu::to_stf(tonemapper); |
|||
} |
|||
} |
|||
|
|||
template <typename C> |
|||
void output(C const & canvas, stfu::node const & stf_input, std::string const & filename, stfu::node & stf_output){ |
|||
if(stf_input.getValue("class") == "colorizer") { |
|||
Tonemappers::Colorizer tonemapper(canvas.layers(), stf_input); |
|||
details::output(canvas, tonemapper, filename, stf_output); |
|||
} |
|||
} |
|||
|
|||
template <typename C> |
|||
void output(C const & canvas, Random::parameters, std::string const & filename, stfu::node & stf_output) { |
|||
Tonemappers::Colorizer tonemapper(canvas.layers(), Random::parameters()); |
|||
details::output(canvas, tonemapper, filename, stf_output); |
|||
} |
|||
|
|||
#endif |
@ -0,0 +1,62 @@ |
|||
//
|
|||
// render.hpp
|
|||
// AwesomeAttract0r
|
|||
//
|
|||
// Created by Joshua Moerman on 1/8/12.
|
|||
// Copyright (c) 2012 Vadovas. All rights reserved.
|
|||
//
|
|||
|
|||
#ifndef AwesomeAttract0r_render_hpp |
|||
#define AwesomeAttract0r_render_hpp |
|||
|
|||
#include <stdexcept> |
|||
|
|||
#include "stf.hpp" |
|||
#include "Logger.hpp" |
|||
#include "Random.hpp" |
|||
#include "Attractor.hpp" |
|||
|
|||
namespace details { |
|||
struct empty_canvas : public std::runtime_error { |
|||
empty_canvas() |
|||
: std::runtime_error("Canvas is too empty (no chaos)") |
|||
{ } |
|||
}; |
|||
|
|||
template <typename C> |
|||
void render(Attractor & myAttractor, C & canvas, unsigned int iterations){ |
|||
Progressbar progress(std::cout, LOG_INFO, "rendering"); |
|||
for(unsigned int j = 1; j <= iterations; ++j) { |
|||
for(unsigned int i = 0; i < 1000000; ++i) { |
|||
myAttractor.iterate(); |
|||
myAttractor.project(); |
|||
canvas.plot(myAttractor.projector->projectedPoint, 0); |
|||
auto c = Random::in_circle(0.3); |
|||
double blur[2] = {myAttractor.projector->projectedPoint[0] + c.first, myAttractor.projector->projectedPoint[1] + c.second}; |
|||
canvas.plot(blur, 1); |
|||
} |
|||
progress.show(j, iterations); |
|||
if(j == iterations/4) if(!filled(canvas, 0.01)) throw empty_canvas(); |
|||
if(j == iterations/4 * 2) if(!filled(canvas, 0.02)) throw empty_canvas(); |
|||
if(j == iterations/4 * 3) if(!filled(canvas, 0.03)) throw empty_canvas(); |
|||
} |
|||
} |
|||
} |
|||
|
|||
template <typename C> |
|||
void render(C & canvas, std::string const & attractorFile, stfu::node & stf_output, unsigned int iterations) { |
|||
Attractor my_attractor(attractorFile); |
|||
|
|||
my_attractor.init_range(); |
|||
|
|||
logger.start("rendering"); |
|||
details::render(my_attractor, canvas, iterations); |
|||
logger.stop(); |
|||
|
|||
if(!filled(canvas, 0.04)) |
|||
throw details::empty_canvas(); |
|||
|
|||
stf_output = stfu::to_stf(my_attractor); |
|||
} |
|||
|
|||
#endif |
Reference in new issue