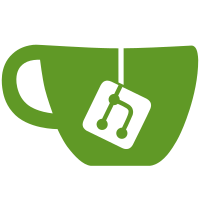
5 changed files with 95 additions and 113 deletions
@ -1,62 +1,10 @@ |
|||||
#include "Logger.hpp" |
#include "Logger.hpp" |
||||
#include "Projector.hpp" |
#include "Projector.hpp" |
||||
|
|
||||
#include <algorithm> |
|
||||
|
|
||||
#include "Canvas.hpp" |
|
||||
|
|
||||
#pragma mark - |
|
||||
#pragma mark memory |
|
||||
|
|
||||
Projector::Projector(unsigned int inputDimension, unsigned int outputDimension) : canvas(0), projector(0), projectedPoint(0), inputDimension(inputDimension), outputDimension(outputDimension), ready(true) { |
|
||||
try { |
|
||||
allocate(); |
|
||||
} catch(std::exception& e) { |
|
||||
LogError("Couldn't construct Projector: %s\n", e.what()); |
|
||||
deallocate(); |
|
||||
} |
|
||||
std::fill_n(projectedPoint, outputDimension, 0.0); |
|
||||
} |
|
||||
|
|
||||
Projector::~Projector() { |
|
||||
deallocate(); |
|
||||
} |
|
||||
|
|
||||
void Projector::allocate() { |
|
||||
projectedPoint = new double[outputDimension]; |
|
||||
} |
|
||||
|
|
||||
void Projector::deallocate() { |
|
||||
delete[] projectedPoint; |
|
||||
projectedPoint = NULL; |
|
||||
} |
|
||||
|
|
||||
#pragma mark - |
|
||||
#pragma mark plot |
|
||||
|
|
||||
void Projector::plot(const double* point) { |
|
||||
project(point); |
|
||||
|
|
||||
if(ready) { |
|
||||
if(canvas != NULL) { |
|
||||
canvas->plot(projectedPoint); |
|
||||
} |
|
||||
|
|
||||
if(projector != NULL) { |
|
||||
projector->plot(projectedPoint); |
|
||||
} |
|
||||
} |
|
||||
} |
|
||||
|
|
||||
#pragma mark - |
|
||||
#pragma mark factory function |
|
||||
|
|
||||
#include "projectors/Normalizer.hpp" |
#include "projectors/Normalizer.hpp" |
||||
|
|
||||
Projector* Projector::createProjector(stfu::node& projector, unsigned int input_dimension) { |
Projector* Projector::createProjector(stfu::node& projector, unsigned int input_dimension) { |
||||
|
|
||||
Projector* output = new Normalizer(input_dimension); |
Projector* output = new Normalizer(input_dimension); |
||||
|
|
||||
return output; |
return output; |
||||
} |
} |
||||
|
|
||||
|
Reference in new issue