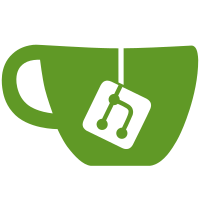
4 changed files with 98 additions and 75 deletions
@ -0,0 +1,87 @@ |
|||
#pragma once |
|||
|
|||
#include "basics.h" |
|||
#include "adaptions.h" |
|||
|
|||
#include "simulation/Simulation.h" |
|||
#include "simulation/Beat.h" |
|||
|
|||
using Vec2 = math::Vec2; |
|||
using LineKind = simulation::LineKind; |
|||
|
|||
using ball_info = int; |
|||
using line_info = void; |
|||
using ball_type = simulation::Ball<ball_info>; |
|||
using line_type = simulation::Line<line_info>; |
|||
using simu_type = simulation::Simulation<ball_info, line_info>; |
|||
|
|||
using note_info = Vec2; |
|||
using note_type = Note<note_info>; |
|||
using beat_type = Beat<note_info>; |
|||
|
|||
struct AbstractLine { |
|||
math::Vec2 starting_point; |
|||
math::Vec2 end_point; |
|||
int line_kind; |
|||
|
|||
const float hsize = 5.0f; |
|||
|
|||
AbstractLine() = default; |
|||
|
|||
AbstractLine(math::Vec2 starting_point, math::Vec2 end_point, int line_kind) |
|||
: starting_point(starting_point) |
|||
, end_point(end_point) |
|||
, line_kind(line_kind) |
|||
{} |
|||
|
|||
// create 6 lines, to emulate width, and rounded edges
|
|||
std::vector<line_type> calculate_lines() const { |
|||
auto dir = normalize(end_point - starting_point); |
|||
auto normal = rotate_ccw(dir); |
|||
LineKind lk = static_cast<LineKind>(line_kind); |
|||
|
|||
if(line_kind == simulation::kOneWay){ |
|||
std::vector<line_type> ret; |
|||
ret.emplace_back(starting_point + hsize*normal, end_point + hsize*normal, lk); |
|||
ret.emplace_back(end_point + hsize*normal, end_point + hsize*dir, lk); |
|||
ret.emplace_back(end_point + hsize*dir, end_point - hsize*normal, lk); |
|||
ret.emplace_back(end_point - hsize*normal, starting_point - hsize*normal, lk); |
|||
ret.emplace_back(starting_point - hsize*normal, starting_point - hsize*dir, lk); |
|||
ret.emplace_back(starting_point - hsize*dir, starting_point + hsize*normal, lk); |
|||
return ret; |
|||
} else { |
|||
std::vector<line_type> ret; |
|||
ret.emplace_back(starting_point, end_point, lk); |
|||
return ret; |
|||
} |
|||
} |
|||
}; |
|||
|
|||
BOOST_FUSION_ADAPT_STRUCT( |
|||
AbstractLine, |
|||
(::math::Vec2, starting_point) |
|||
(::math::Vec2, end_point) |
|||
(int, line_kind) |
|||
) |
|||
|
|||
BOOST_FUSION_DEFINE_STRUCT( |
|||
, IntVec2, |
|||
(int, x) |
|||
(int, y) |
|||
) |
|||
|
|||
struct cheap_ball_type { |
|||
IntVec2 position; |
|||
ball_info information; |
|||
|
|||
cheap_ball_type(ball_type const & b) |
|||
: position(b.position) |
|||
, information(b.information) |
|||
{} |
|||
}; |
|||
|
|||
BOOST_FUSION_ADAPT_STRUCT( |
|||
cheap_ball_type, |
|||
(IntVec2, position) |
|||
(ball_info, information) |
|||
) |
Reference in new issue