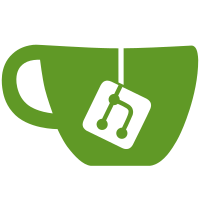
9 changed files with 201 additions and 51 deletions
@ -1,2 +1,2 @@ |
|||
#!/bin/bash |
|||
while true; do ./AwesomeAttractor -P /var/www/render2/ -R -I 2000 -W 1024 -H 1024 -v attractors/emptyUnravel3D.stf; done |
|||
while true; do ~/Library/Developer/Xcode/DerivedData/AwesomeAttract0r-akxvgtneyociakezlpkfgaywuvoo/Build/Products/Debug/AwesomeAttract0r -P /Users/joshua/Documents/Code/AwesomeAttractor/render/ -R -I 20 -W 640 -H 640 -v attractors/emptyUnravel3D.stf; done |
|||
|
@ -0,0 +1,88 @@ |
|||
//
|
|||
// bmp.hpp
|
|||
// AwesomeAttract0r
|
|||
//
|
|||
// Created by Joshua Moerman on 10/24/11.
|
|||
// Copyright 2011 Vadovas. All rights reserved.
|
|||
//
|
|||
|
|||
#ifndef AwesomeAttract0r_bmp_hpp |
|||
#define AwesomeAttract0r_bmp_hpp |
|||
|
|||
#include <iostream> |
|||
#include <fstream> |
|||
#include <algorithm> |
|||
#include <iterator> |
|||
|
|||
namespace bmp { |
|||
|
|||
struct bitmap_file_header { |
|||
uint32_t filesize; |
|||
uint16_t creator1; |
|||
uint16_t creator2; |
|||
uint32_t bmp_offset; |
|||
|
|||
template <typename DIBT> |
|||
bitmap_file_header(DIBT dib_header): |
|||
filesize(dib_header.bytes() + dib_header.header_sz + 12 + 2), |
|||
creator1(0), |
|||
creator2(0), |
|||
bmp_offset(dib_header.header_sz + 12 + 2){} |
|||
|
|||
void write(std::ostream& out) const { |
|||
out << "BM"; |
|||
out.write(reinterpret_cast<const char*>(this), 12); |
|||
} |
|||
}; |
|||
|
|||
struct bitmapcoreheader { |
|||
uint32_t header_sz; |
|||
uint16_t width; |
|||
uint16_t height; |
|||
uint16_t nplanes; |
|||
uint16_t bitspp; |
|||
|
|||
bitmapcoreheader(int width, int height): |
|||
header_sz(sizeof(bitmapcoreheader)), |
|||
width(width), |
|||
height(height), |
|||
nplanes(1), |
|||
bitspp(24){} |
|||
|
|||
void write(std::ostream& out) const { |
|||
out.write(reinterpret_cast<const char*>(this), header_sz); |
|||
} |
|||
|
|||
unsigned int bytes(){ |
|||
return width*height*bitspp/8; |
|||
} |
|||
}; |
|||
|
|||
template <typename T, typename DIBT = bitmapcoreheader> |
|||
struct bitmap { |
|||
DIBT dib_header; |
|||
bitmap_file_header header; |
|||
T const * data; |
|||
|
|||
bitmap(int width, int height) |
|||
: dib_header(width, height) |
|||
, header(dib_header) |
|||
, data(0) {} |
|||
|
|||
void write(std::string const & filename){ |
|||
std::ofstream file(filename.c_str()); |
|||
write(file); |
|||
} |
|||
|
|||
void write(std::ostream& out){ |
|||
header.write(out); |
|||
dib_header.write(out); |
|||
//std::copy_n((char const *)data, dib_header.bytes(), std::ostream_iterator<char>(out));
|
|||
std::copy((char const *)data, (char const *)data + dib_header.bytes(), std::ostream_iterator<char>(out)); |
|||
} |
|||
|
|||
}; |
|||
|
|||
} |
|||
|
|||
#endif |
Reference in new issue