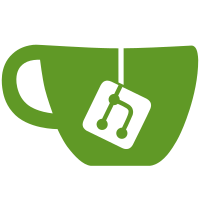
11 changed files with 78 additions and 370 deletions
@ -1,110 +0,0 @@ |
|||||
#include <iostream> |
|
||||
using namespace std; |
|
||||
|
|
||||
#include "Parameters.hpp" |
|
||||
|
|
||||
|
|
||||
Parameters::Parameters(unsigned int num_parameters, float default_val): |
|
||||
num_parameters(num_parameters) { |
|
||||
begin = new (nothrow) Vector(num_parameters, default_val); |
|
||||
eind = new (nothrow) Vector(num_parameters, default_val); |
|
||||
interpolated = new (nothrow) Vector(num_parameters, default_val); |
|
||||
// *interpolated = *begin
|
|
||||
|
|
||||
check_pointers(); |
|
||||
|
|
||||
#ifdef HARDDEBUG |
|
||||
cout << "New Parameters with one default val:" << endl << *this << endl; |
|
||||
#endif |
|
||||
} |
|
||||
|
|
||||
/*Parameters::Parameters(unsigned int num_parameters, float default_val1, float default_val2):
|
|
||||
num_parameters(num_parameters) { |
|
||||
begin = new (nothrow) Vector(num_parameters, default_val1); |
|
||||
eind = new (nothrow) Vector(num_parameters, default_val2); |
|
||||
interpolated = new (nothrow) Vector(num_parameters, default_val1); |
|
||||
// *interpolated = *begin
|
|
||||
|
|
||||
check_pointers(); |
|
||||
|
|
||||
#ifdef HARDDEBUG |
|
||||
cout << "New Parameters with two default vals:" << endl << *this << endl; |
|
||||
#endif |
|
||||
}*/ |
|
||||
|
|
||||
Parameters::~Parameters() { |
|
||||
delete begin; |
|
||||
delete eind; |
|
||||
delete interpolated; |
|
||||
|
|
||||
#ifdef HARDDEBUG |
|
||||
cout << "Parameters deleted" << endl; |
|
||||
#endif |
|
||||
} |
|
||||
|
|
||||
void Parameters::check_pointers() { |
|
||||
assert(begin != NULL); |
|
||||
assert(eind != NULL); |
|
||||
assert(interpolated != NULL); |
|
||||
} |
|
||||
|
|
||||
void Parameters::set(unsigned int parameter, float val1, float val2) { |
|
||||
assert(parameter < num_parameters); |
|
||||
|
|
||||
begin->coordinates[parameter] = val1; |
|
||||
eind->coordinates[parameter] = val2; |
|
||||
interpolated->coordinates[parameter] = val1; |
|
||||
|
|
||||
#ifdef HARDDEBUG |
|
||||
cout << "Parameter " << parameter << " set to: " << val1 << " - " << val2 << endl; |
|
||||
#endif |
|
||||
} |
|
||||
|
|
||||
void Parameters::set(unsigned int parameter, float val) { |
|
||||
assert(parameter < num_parameters); |
|
||||
|
|
||||
begin->coordinates[parameter] = val; |
|
||||
eind->coordinates[parameter] = val; |
|
||||
interpolated->coordinates[parameter] = val; |
|
||||
|
|
||||
#ifdef HARDDEBUG |
|
||||
cout << "Parameter " << parameter << " set to: " << val << endl; |
|
||||
#endif |
|
||||
} |
|
||||
|
|
||||
float Parameters::get(unsigned int parameter) { |
|
||||
assert(parameter < num_parameters); |
|
||||
|
|
||||
#ifdef HARDDEBUG |
|
||||
cout << "Asked for parameter " << parameter << " with value:" << interpolated->coordinates[parameter] << endl; |
|
||||
#endif |
|
||||
|
|
||||
return interpolated->coordinates[parameter]; |
|
||||
|
|
||||
} |
|
||||
|
|
||||
void Parameters::interpolate(float time) { |
|
||||
/*
|
|
||||
Dit is mogelijk met vector rekenen: |
|
||||
(*interpolated) = (*begin) * ( 1.0 - time ) + (*eind) * time; |
|
||||
Maar we doen het per element, zodat we simpelere code hebben, |
|
||||
geen vectoren hoeven te returnen en makkelijker kunnen optimaliseren |
|
||||
*/ |
|
||||
const float invtime = 1.0 - time; |
|
||||
for ( unsigned int i = 0; i < num_parameters; i++ ) { |
|
||||
interpolated->coordinates[i] = invtime * begin->coordinates[i] + time * eind->coordinates[i]; |
|
||||
} |
|
||||
|
|
||||
#ifdef HARDDEBUG |
|
||||
cout << "interpolate() result" << endl << *interpolated << endl; |
|
||||
#endif |
|
||||
} |
|
||||
|
|
||||
ostream& operator<<(ostream& os, const Parameters& param) { |
|
||||
os << param.num_parameters << endl; |
|
||||
os << "Begin:" << endl << *param.begin << endl; |
|
||||
os << "Eind:" << endl << *param.eind << endl; |
|
||||
os << "Interpolated:" << endl << *param.interpolated << endl; |
|
||||
os <<endl; |
|
||||
return os; |
|
||||
} |
|
@ -1,34 +0,0 @@ |
|||||
#ifndef PARAMETER_HPP |
|
||||
#define PARAMETER_HPP |
|
||||
|
|
||||
#include "Vector.hpp" |
|
||||
|
|
||||
class Parameters { |
|
||||
|
|
||||
Vector * begin; |
|
||||
Vector * eind; |
|
||||
Vector * interpolated; |
|
||||
|
|
||||
void check_pointers(); |
|
||||
|
|
||||
|
|
||||
public: |
|
||||
|
|
||||
// for checks and assertions
|
|
||||
unsigned int num_parameters; |
|
||||
|
|
||||
Parameters(unsigned int num_parameters, float default_val = 0.0); |
|
||||
//Parameters(unsigned int num_parameters, float default_val1, float default_val2);
|
|
||||
~Parameters(); |
|
||||
|
|
||||
void set(unsigned int parameter, float val1, float val2); |
|
||||
void set(unsigned int parameter, float val); |
|
||||
float get(unsigned int parameter); |
|
||||
|
|
||||
void interpolate(float time); |
|
||||
|
|
||||
// output operator
|
|
||||
friend ostream& operator<<(ostream& os, const Parameters& param); |
|
||||
}; |
|
||||
|
|
||||
#endif // PARAMETER_HPP
|
|
@ -1,126 +0,0 @@ |
|||||
#include <iostream> |
|
||||
using namespace std; |
|
||||
|
|
||||
#include "Vector.hpp" |
|
||||
|
|
||||
Vector::Vector(): |
|
||||
dimension(0) { |
|
||||
|
|
||||
coordinates = new (nothrow) float[0]; |
|
||||
|
|
||||
assert(coordinates != NULL); |
|
||||
|
|
||||
#ifdef HARDDEBUG |
|
||||
cout << "New vector (without elements)" << endl; |
|
||||
#endif |
|
||||
} |
|
||||
|
|
||||
Vector::Vector(unsigned int d): |
|
||||
dimension(d) { |
|
||||
|
|
||||
coordinates = new (nothrow) float[dimension]; |
|
||||
|
|
||||
assert(coordinates != NULL); |
|
||||
|
|
||||
#ifdef HARDDEBUG |
|
||||
cout << "New vector:" << endl << *this << endl; |
|
||||
#endif |
|
||||
} |
|
||||
|
|
||||
Vector::Vector(unsigned int d, float default_val): |
|
||||
dimension(d) { |
|
||||
|
|
||||
coordinates = new (nothrow) float[dimension]; |
|
||||
|
|
||||
assert(coordinates != NULL); |
|
||||
|
|
||||
for (unsigned int i = 0; i < dimension; i++) { |
|
||||
coordinates[i] = default_val; |
|
||||
} |
|
||||
|
|
||||
#ifdef HARDDEBUG |
|
||||
cout << "New vector with default values:" << endl << *this << endl; |
|
||||
#endif |
|
||||
} |
|
||||
|
|
||||
|
|
||||
Vector::~Vector() { |
|
||||
delete[] coordinates; |
|
||||
|
|
||||
#ifdef HARDDEBUG |
|
||||
cout << "coordinates deleted" << endl; |
|
||||
#endif |
|
||||
} |
|
||||
|
|
||||
|
|
||||
Vector& Vector::operator=(const Vector& a) { |
|
||||
if ( dimension != a.dimension ) { |
|
||||
dimension = a.dimension; |
|
||||
delete[] coordinates; |
|
||||
coordinates = new float[dimension]; |
|
||||
|
|
||||
#ifdef HARDDEBUG |
|
||||
cout << "Dimensions were not equal, made new vector" << endl; |
|
||||
#endif |
|
||||
} |
|
||||
|
|
||||
for ( unsigned int i = 0; i < dimension; i++ ) { |
|
||||
coordinates[i] = a.coordinates[i]; |
|
||||
} |
|
||||
|
|
||||
#ifdef HARDDEBUG |
|
||||
cout << "operator= result" << endl << *this << endl; |
|
||||
#endif |
|
||||
|
|
||||
return *this; |
|
||||
} |
|
||||
|
|
||||
|
|
||||
ostream& operator<<(ostream& os, const Vector& a) { |
|
||||
os << a.dimension << endl; |
|
||||
for ( unsigned int i = 0; i < a.dimension; i++ ) { |
|
||||
os << a.coordinates[i] << " "; |
|
||||
} |
|
||||
|
|
||||
os << endl; |
|
||||
return os; |
|
||||
} |
|
||||
|
|
||||
|
|
||||
float& Vector::operator[](const unsigned int index) { |
|
||||
assert(index < dimension); |
|
||||
return coordinates[index]; |
|
||||
} |
|
||||
|
|
||||
// matig werkende optelling en scalaire vermenigvuldiging van vectoren
|
|
||||
/*
|
|
||||
Vector Vector::operator+(const Vector a) const { |
|
||||
if ( dimension != a.dimension ) { |
|
||||
cout << "WARNING: dimensions not equal in vector addition" << endl; |
|
||||
exit(1); |
|
||||
} else { |
|
||||
static Vector ret(dimension); |
|
||||
for ( unsigned int i = 0; i < dimension; i++ ) { |
|
||||
ret.coordinates[i] = coordinates[i] + a.coordinates[i]; |
|
||||
} |
|
||||
|
|
||||
#ifdef HARDDEBUG |
|
||||
cout << "operator+ result" << endl << ret << endl; |
|
||||
#endif |
|
||||
return ret; |
|
||||
} |
|
||||
} |
|
||||
|
|
||||
|
|
||||
Vector Vector::operator*(const float a) const { |
|
||||
static Vector ret(dimension); |
|
||||
for ( unsigned int i = 0; i < dimension; i++ ) { |
|
||||
ret.coordinates[i] = coordinates[i] * a; |
|
||||
} |
|
||||
|
|
||||
#ifdef HARDDEBUG |
|
||||
cout << "operator* result" << endl << ret << endl; |
|
||||
#endif |
|
||||
return ret; |
|
||||
} |
|
||||
*/ |
|
@ -1,31 +0,0 @@ |
|||||
#ifndef VECTOR_HPP |
|
||||
#define VECTOR_HPP |
|
||||
|
|
||||
class Vector { |
|
||||
public: |
|
||||
|
|
||||
unsigned int dimension; |
|
||||
float * coordinates; |
|
||||
|
|
||||
// const, dest
|
|
||||
Vector(); |
|
||||
Vector(unsigned int d); |
|
||||
Vector(unsigned int d, float default_val); |
|
||||
~Vector(); |
|
||||
|
|
||||
// output operator
|
|
||||
friend ostream& operator<<(ostream& os, const Vector& a); |
|
||||
|
|
||||
// easy access
|
|
||||
float& Vector::operator[](const unsigned int index); |
|
||||
|
|
||||
// vector rekenen
|
|
||||
Vector& operator=(const Vector& a); |
|
||||
// faaloperatoren
|
|
||||
// Vector operator+(const Vector a) const;
|
|
||||
// Vector operator*(const float a) const;
|
|
||||
}; |
|
||||
|
|
||||
|
|
||||
#endif // VECTOR_HPP
|
|
||||
|
|
@ -1,15 +1,13 @@ |
|||||
|
|
||||
|
#define DEFAULT_ATTRACTOR_FILE "attractors/testUnravel.stf" |
||||
//TODO: do this with files
|
|
||||
#define ATTRACTOR_FILE "attractors/testUnravel.stf" |
|
||||
|
|
||||
#ifdef UNI_BUILD |
#ifdef UNI_BUILD |
||||
#warning Building for the RU, are you sure? |
#warning Building for the RU, are you sure? |
||||
#define WIDTH 8000 |
#define DEFAULT_WIDTH 8000 |
||||
#define HEIGHT 8000 |
#define DEFAULT_HEIGHT 8000 |
||||
#define ITERATIONS 4200000000 |
#define DEFAULT_ITERATIONS 4200000000 |
||||
#else |
#else |
||||
#define WIDTH 800 |
#define DEFAULT_WIDTH 800 |
||||
#define HEIGHT 800 |
#define DEFAULT_HEIGHT 800 |
||||
#define ITERATIONS 1000000 |
#define DEFAULT_ITERATIONS 1000000 |
||||
#endif |
#endif |
||||
|
Reference in new issue