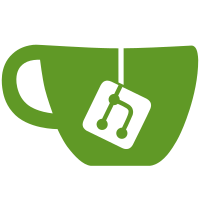
5 changed files with 111 additions and 143 deletions
@ -0,0 +1,7 @@ |
|||
#!/bin/bash |
|||
|
|||
mkdir -p build |
|||
cd build |
|||
cmake .. |
|||
make -j1 |
|||
cd .. |
@ -0,0 +1,75 @@ |
|||
|
|||
#include "websockets.h" |
|||
|
|||
namespace websockets { |
|||
|
|||
option options[] = { |
|||
{ "help", no_argument, NULL, 'h' }, |
|||
{ "port", required_argument, NULL, 'p' }, |
|||
{ "ssl", no_argument, NULL, 's' }, |
|||
{ "interface", required_argument, NULL, 'i' }, |
|||
{ NULL, 0, 0, 0 } |
|||
}; |
|||
|
|||
int default_main(int argc, char **argv, libwebsocket_protocols* protocols){ |
|||
int port = 7681; |
|||
int use_ssl = 0; |
|||
char interface_name[128] = ""; |
|||
const char *interface = NULL; |
|||
|
|||
int n = 0; |
|||
while (n >= 0) { |
|||
n = getopt_long(argc, argv, "i:hsp:", options, NULL); |
|||
if (n < 0) continue; |
|||
|
|||
switch (n) { |
|||
case 's': |
|||
use_ssl = 1; /* 1 = take care about cert verification, 2 = allow anything */ |
|||
break; |
|||
case 'p': |
|||
port = atoi(optarg); |
|||
break; |
|||
case 'i': |
|||
strncpy(interface_name, optarg, sizeof interface_name); |
|||
interface_name[(sizeof interface_name) - 1] = '\0'; |
|||
interface = interface_name; |
|||
break; |
|||
case '?': |
|||
case 'h': |
|||
fprintf(stderr, "Usage: libwebsockets-test-echo [--ssl] [--port=<p>] [--interface=iface]"); |
|||
exit(1); |
|||
} |
|||
} |
|||
|
|||
websockets::Log log("lwsts", LOG_PID | LOG_PERROR, 7); |
|||
log.notice("Running in server mode\n"); |
|||
|
|||
lws_context_creation_info info; |
|||
memset(&info, 0, sizeof info); |
|||
|
|||
info.port = port; |
|||
info.iface = interface; |
|||
info.protocols = protocols; |
|||
info.gid = -1; |
|||
info.uid = -1; |
|||
|
|||
if (use_ssl) { |
|||
info.ssl_cert_filepath = "libwebsockets-test-server.pem"; |
|||
info.ssl_private_key_filepath = "libwebsockets-test-server.key.pem"; |
|||
} |
|||
|
|||
websockets::Context context(info); |
|||
|
|||
static bool force_exit = false; |
|||
signal(SIGINT, [](int){ force_exit = true; }); |
|||
|
|||
while (!force_exit) { |
|||
auto ret = libwebsocket_service(context.get_raw(), 10); |
|||
if(ret < 0) break; |
|||
} |
|||
|
|||
log.notice("libwebsockets-test-echo exited cleanly\n"); |
|||
return 0; |
|||
} |
|||
|
|||
} // namespace websockets
|
Reference in new issue