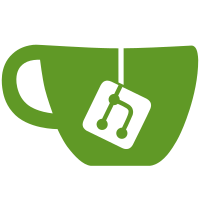
1 changed files with 91 additions and 0 deletions
@ -0,0 +1,91 @@ |
|||
//
|
|||
// progressbar.hpp
|
|||
//
|
|||
// Created by Joshua Moerman on 11/5/11.
|
|||
// Copyright 2011 Vadovas. All rights reserved.
|
|||
//
|
|||
|
|||
/*
|
|||
A simple progressbar for the terminal (relies on \r). |
|||
Wrap your for loop in an extra scope with a progressbar, like this: |
|||
|
|||
{ // extra scope
|
|||
Progressbar p(std::cout); |
|||
for(i = 0; i < 1000; ++i){ |
|||
... |
|||
p.show(i, 999); |
|||
} |
|||
} // end extra scope (destructor of Progressbar will be used).
|
|||
|
|||
You can give extra information to show in the constructor: |
|||
Progressbar p(stream, "prefix", "Start message", " End message"); |
|||
this will look like: |
|||
|
|||
Start message |
|||
prefix [===============> ] |
|||
End message |
|||
|
|||
You may leave these things empty (""). |
|||
|
|||
NOTE: the width of the bar (incl. prefix) is fixed ATM. |
|||
NOTE: pass the maximum-value to the function show, not the bound of the for-loop (this way the function also works nicely for floating types) |
|||
*/ |
|||
|
|||
#ifndef PROGRESSBAR_HPP |
|||
#define PROGRESSBAR_HPP |
|||
|
|||
#include <iostream> |
|||
|
|||
struct progressbar { |
|||
progressbar(std::ostream & out, std::string prefix = "", std::string begin = "", std::string end = "") |
|||
: out(out) |
|||
, begin(begin) |
|||
, prefix(prefix) |
|||
, end(end) |
|||
{ |
|||
if (begin != "") { |
|||
out << begin << "\n"; |
|||
} |
|||
|
|||
show(0, 1, ' '); |
|||
} |
|||
|
|||
~progressbar(){ |
|||
show(1, 1, '='); |
|||
|
|||
if (end != "") { |
|||
out << "\n" << end << "\n"; |
|||
} else { |
|||
out << "\n"; |
|||
} |
|||
} |
|||
|
|||
template <typename T> |
|||
void show(T const & progress, T const & max, char delim = '>'){ |
|||
out << "\r"; |
|||
|
|||
size_t width = 80; // default terminal size :D
|
|||
width -= prefix.size(); |
|||
width -= 3; // [, > and ]
|
|||
|
|||
if (prefix != "") { |
|||
width -= 1; |
|||
out << prefix << ' '; |
|||
} |
|||
|
|||
double ratio = (double) progress / (double) max; |
|||
size_t length = width * ratio; |
|||
|
|||
std::string fill(length, '='); |
|||
std::string empty(width - length, ' '); |
|||
out << '[' << fill << delim << empty << ']'; |
|||
} |
|||
|
|||
private: |
|||
std::ostream & out; |
|||
std::string begin; |
|||
std::string prefix; |
|||
std::string end; |
|||
}; |
|||
|
|||
#endif // PROGRESSBAR_HPP
|
Reference in new issue