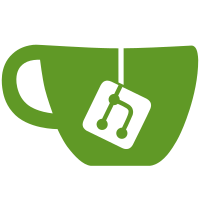
3 changed files with 115 additions and 15 deletions
@ -0,0 +1,48 @@ |
|||||
|
module Automaton exposing (..) |
||||
|
|
||||
|
import Random |
||||
|
|
||||
|
-- MODEL |
||||
|
|
||||
|
type alias Automaton s i = List ((s, i), s) |
||||
|
|
||||
|
zip : List a -> List b -> List (a, b) |
||||
|
zip = List.map2 (,) |
||||
|
|
||||
|
cart : List a -> List b -> List (a, b) |
||||
|
cart l1 l2 = List.concat <| List.map (\x -> List.map (\y -> (x, y)) l2) l1 |
||||
|
|
||||
|
genAut : Int -> Int -> Random.Generator (Automaton Int Int) |
||||
|
genAut n k = let |
||||
|
allSI = cart [1..n] [1..k] |
||||
|
in Random.map (zip allSI) (Random.list (n*k) <| Random.int 1 n) |
||||
|
|
||||
|
step : Automaton s i -> Maybe s -> i -> Maybe s |
||||
|
step ls s0 i = case s0 of |
||||
|
Nothing -> Nothing |
||||
|
Just s -> List.filter (\((t, j), b) -> t == s && i == j) ls |> List.head |> Maybe.map snd |
||||
|
|
||||
|
--(=>) = (,) |
||||
|
|
||||
|
--state2Color : State -> Color |
||||
|
--state2Color s = case s of |
||||
|
-- Nothing -> Color.black |
||||
|
-- Just n -> hsl (degrees (toFloat n)*77) 1.0 (0.8 - 0.6 * (toFloat n)/size) |
||||
|
|
||||
|
--color2String : Color -> String |
||||
|
--color2String c = let rgb = toRgb c in "rgb(" ++ toString rgb.red ++ ", " ++ toString rgb.green ++ ", " ++ toString rgb.blue ++ ")" |
||||
|
|
||||
|
--block : Color -> Html msg |
||||
|
--block c = div [style ["background-color" => color2String c, "width" => "100px", "height" => "100px", "display" => "inline-block"]] [] |
||||
|
|
||||
|
--view : Model -> Html Msg |
||||
|
--view model = |
||||
|
-- div [] |
||||
|
-- ( button [ onClick Regen ] [ text "Regenerate" ] |
||||
|
-- :: button [ onClick Sort ] [ text "Sort" ] |
||||
|
-- :: br [] [] |
||||
|
-- :: List.map (block << state2Color) model.states |
||||
|
-- ) |
||||
|
|
||||
|
|
||||
|
|
Reference in new issue